SOAP over WebSockets and InfiniBand with JAX-WS Pluggable Transports
My presentation at JavaOne 2013.
ABSTRACT
The JAX-WS standard includes APIs for using POJOs or XML for remote messages. But it does not include APIs for letting the user control the transport. This BoF discusses adding pluggable transport APIs to the JAX-WS standard.
This BoF shows a candidate pluggable transport mechanism for JAX-WS that enables one to use other transports besides HTTP. In particular, it shows the benefits of using WebSockets and InfiniBand transports for SOAP message exchanges.
SUMMARY
Background:
Besides being able to send and receive remote method calls with Java objects (POJOs), JAX-WS has APIs that enable one to operate at the XML level. “Dispatch” is available on the client-side to feed XML into JAX-WS for soap-processing (e.g., handling security). The message is then sent using the transport built into the system. After the response is received and processed, the XML is given back to Dispatch. Dispatch also has an asynchronous mode.
The service-side has a similar “Provider” API, except Provider does not have an asynchronous mode. However, the JAX-WS specification does not have corresponding APIs for giving the user control of the transport in a similar manner.
Pluggable Transports:
Asynchronous APIs for client and service transport are shown, as well as APIs for plugging those transports into the underlying JAX-WS system. Using those APIs, the BoF includes a demonstration of plugging in WebSocket, InfiniBand and RDMA transports into an existing system.
The benefits of these transports are shown, such as:
- the ability to use an alternate transport if the vendor does not supply it
- ability to use binary encodings
- long lasting connections
- performance (with numbers from production environments)
- direct, application-to-application, zero-copy memory access that by-passes the operating system
The benefits are not without drawbacks, such as:
- connection management is now the responsibility of the transport writer instead of the platform
- memory management for zero-copy
These APIs have been used in a production environment.
me, disclaimer, copyright
BOF7479 : SOAP over WebSockets and InfiniBand with JAX-WS Pluggable Transports
Harold Carr
architect of SOAP Web Services Technology at Oracle
Last Modified : 2013 Dec 15 (Sun) 13:15:19 by carr.
disclaimer, copyright
THE FOLLOWING IS INTENDED TO OUTLINE OUR GENERAL PRODUCT DIRECTION. IT IS INTENDED FOR INFORMATION PURPOSES ONLY, AND MAY NOT BE INCORPORATED INTO ANY CONTRACT. IT IS NOT A COMMITMENT TO DELIVER ANY MATERIAL, CODE, OR FUNCTIONALITY, AND SHOULD NOT BE RELIED UPON IN MAKING PURCHASING DECISIONS. THE DEVELOPMENT, RELEASE, AND TIMING OF ANY FEATURES OR FUNCTIONALITY DESCRIBED FOR ORACLE’S PRODUCTS REMAINS AT THE SOLE DISCRETION OF ORACLE.
- Copyright 2013 Oracle and/or its affiliates.
intro
what you will learn
client APIs to enter/exit JAX-WS
for request processing
- enter:
DispatcherRequest
; exit:ClientRequestTransport
- enter:
for response processing
- enter:
ClientResponseTransport
; exit:DispatcherResponse
- enter:
service APIs to enter/exit JAX-WS
for request processing
- enter:
ServiceRequestTransport
; exit:ProviderRequest
- enter:
for response processing
- enter:
ProviderResponse
; exit:ServiceResponseTransport
- enter:
example dual HTTP/WebSocket transport (uses JSR-356)
potential InfiniBand transport
you will not learn WebSocket nor InfiniBand in detail
motivation: SOAP passing thru intermediary
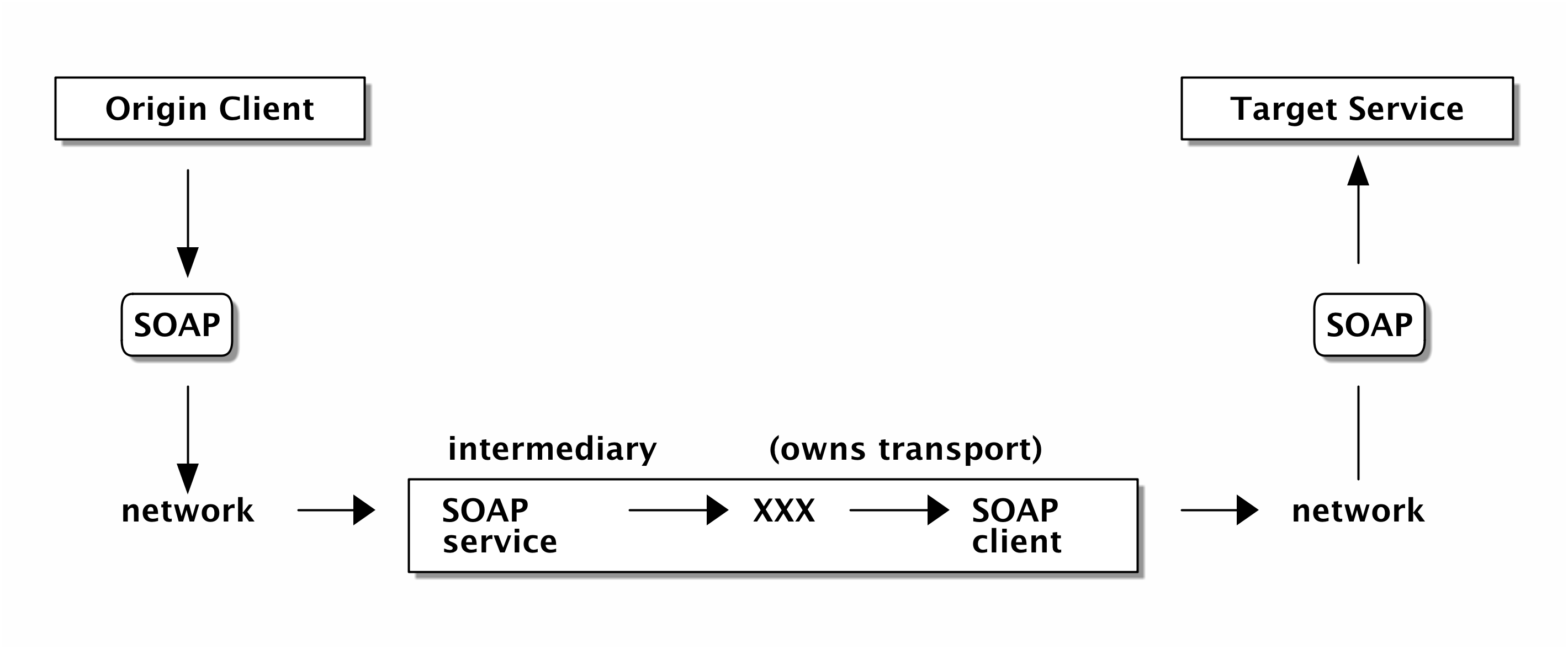
JAX-WS POJO usage
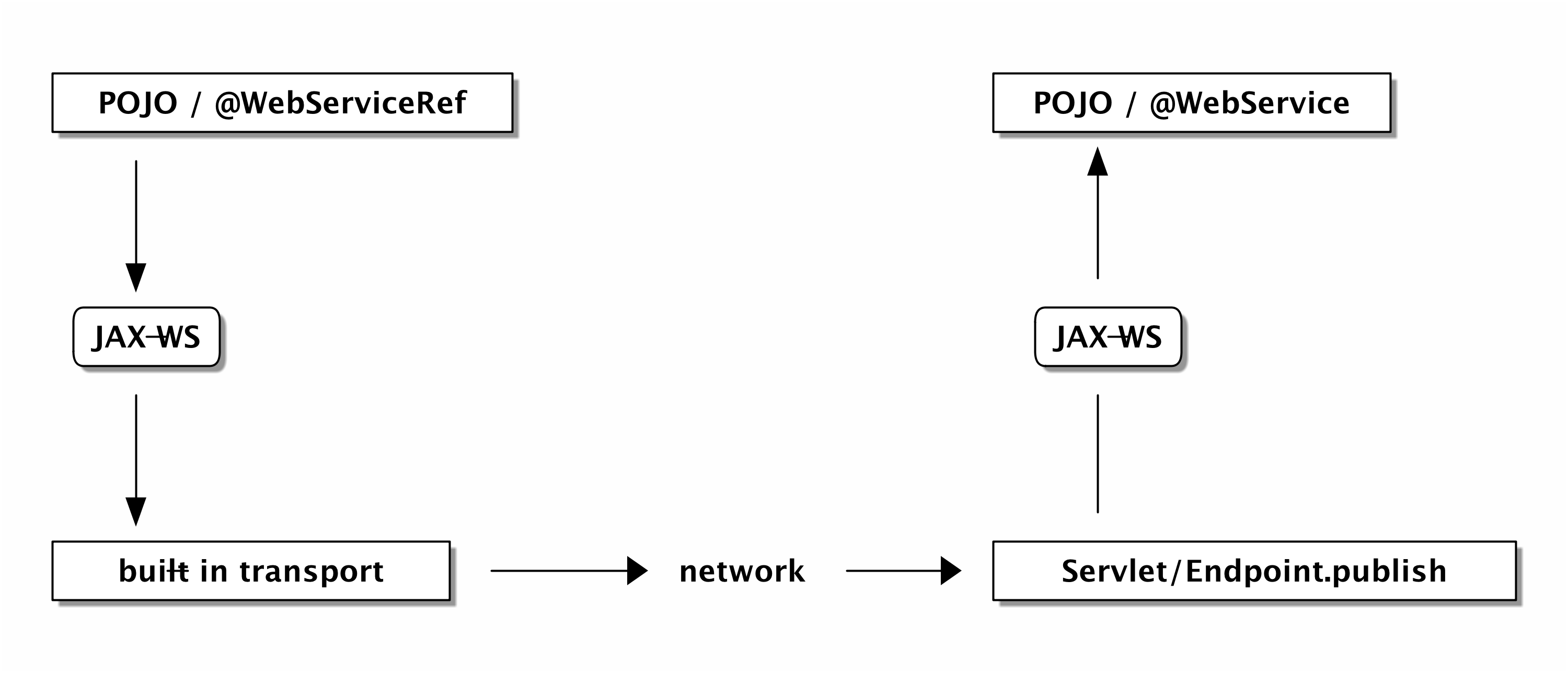
JAX-WS XML usage
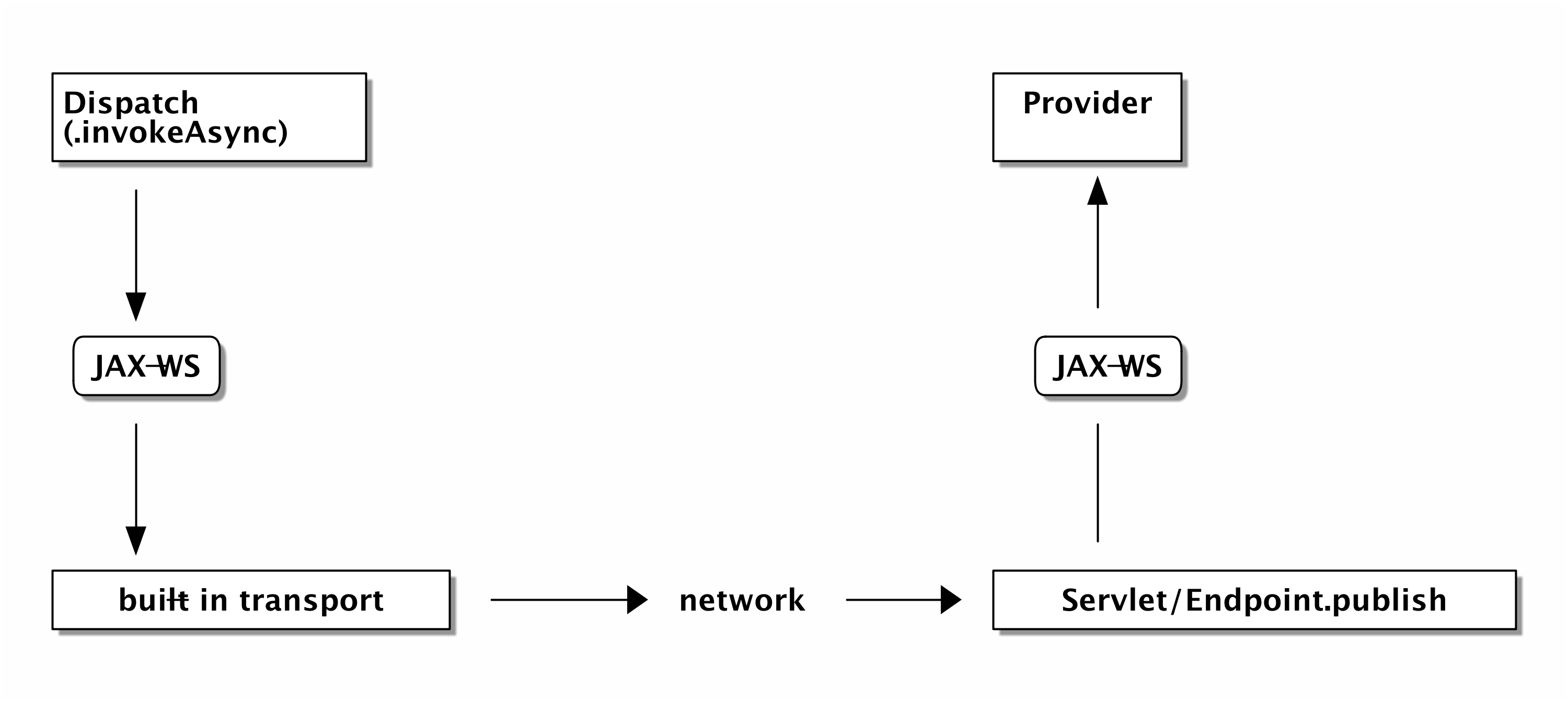
JAX-WS standard includes/lacks
JAX-WS standard
includes APIs for
POJO :
@WebServiceRef
,@WebService
, …XML :
Dispatch
,Provider
, …async :
Dispatch.invokeAsyc
does not include APIs
user transport :
WebSockets
,InfiniBand
, …async everywhere : async
Provider
, …thread guarantees
propose
adding pluggable async transport APIs
adding async
Dispatch
andProvider
thread guarantees
proposal
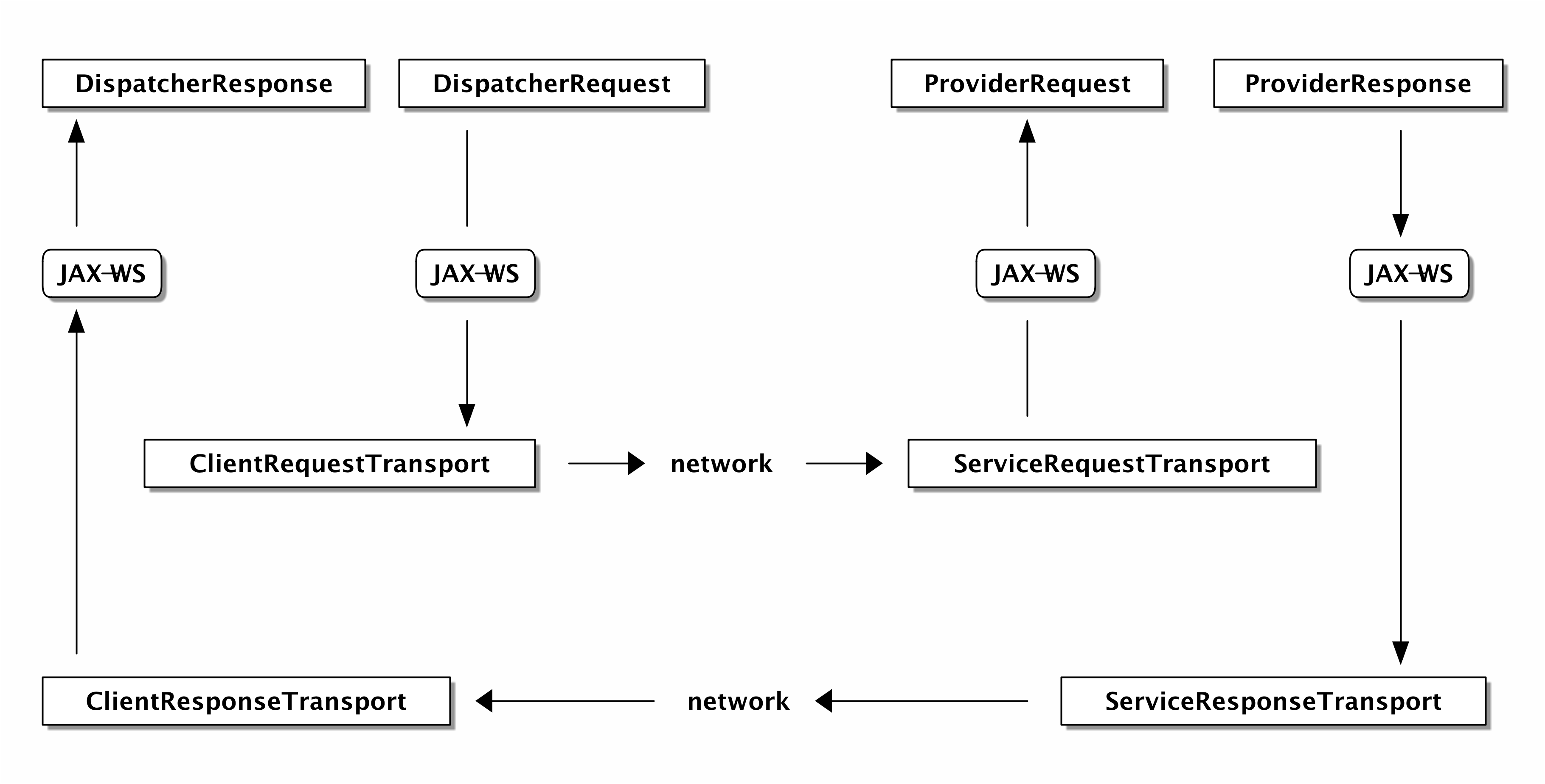
benefits
ability to use alternate transports
e.g., not supplied by vendor/implementation
performance
- e.g., InfiniBand direct, app-to-app, zero-copy memory access by-passes OS
use “last minute” binary encodings
long lasting connections
Drawbacks
connection management responsibility of transport impl instead of platform
memory management for zero-copy
programming with existing JAX-WS
POJO example
@WebService
public class Hello {
@Resource
protected WebServiceContext context;
@WebMethod
public String hello(String name) { ... }
}
public class HelloClient {
@WebServiceRef(wsdlLocation="...?wsdl")
HelloService service;
...
final Hello port = service.getHelloPort();
((BindingProvider)port).getRequest/ResponseContext();
final String response = port.hello(av[0]);
POJO PROS/CONS
PROS
easy to use/deploy
client
port
/BindingProvider
access to request/response contextservice access to request context
CONS
client
context.put
sticky : not per-requestno access to response context on service side
entire XML marshaled to/from POJOs
not async
no access to XML
no thread guarantees
cannot “own” transport
Dispatch
/ Provider
example
@ServiceMode(value=Service.Mode.PAYLOAD) // MESSAGE
@WebServiceProvider(serviceName="HelloService", portName="HelloPort", ...)
public class HelloImpl implements Provider<Source> {
@Resource
protected WebServiceContext context;
public Source invoke(Source source) { ... } }
public class HelloClient {
@WebServiceRef(wsdlLocation="...?wsdl")
HelloService service;
...
Dispatch<Source> d = // SOAPMessage
service.createDispatch(portQName, Source.class,
Service.Mode.PAYLOAD);
Map<String, Object> c = d.getRequest/ResponseContext();
Source result = d.invoke(...); // .invokeAsync
Dispatch
/ Provider
PROS/CONS
PROS
access to XML
Dispatch
has asyncaccess to context
CONS
no
Provider
asyncno response context access in
Provider
Dispatch
/BindingProvider
response context decoupled fromAsyncHandler
no thread guarantees
cannot “own” transport
only
Source
andSOAPMessage
supported- want
InputStream
too
- want
Dynamic Invocation and Service Interfaces (DISI)
DISI client side
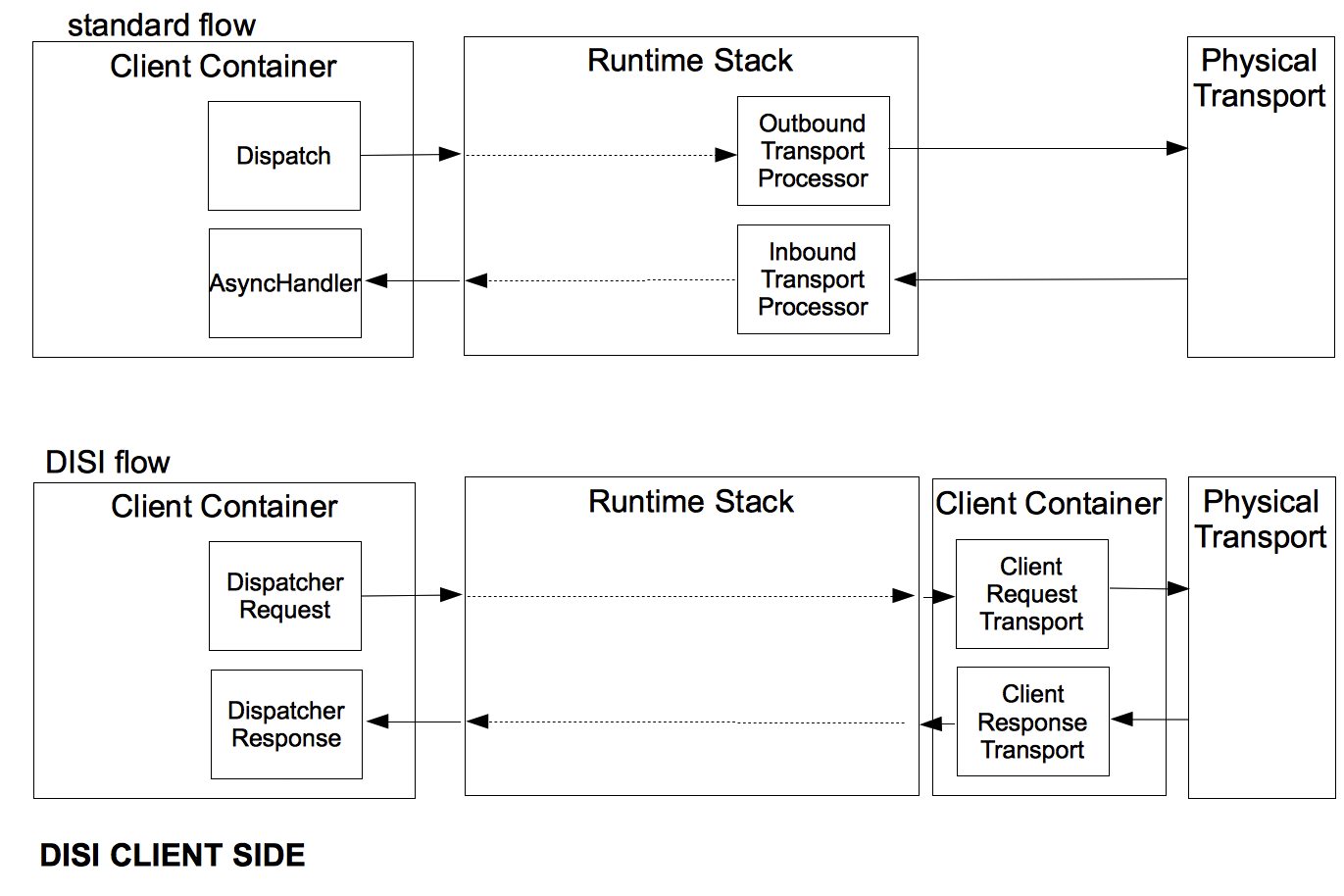
DISI service side
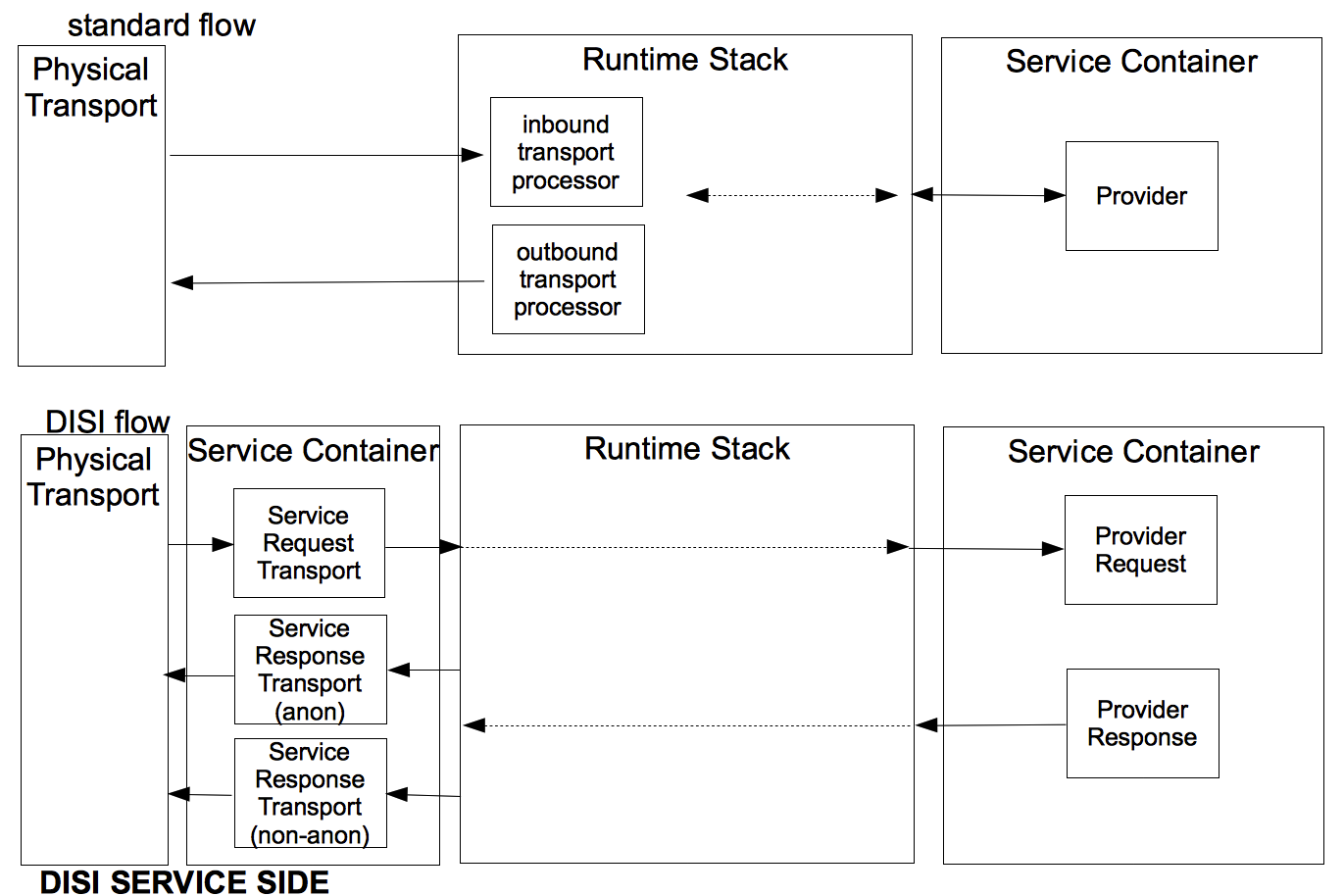
DISI responsibilities

WebSockets transport
simple WebSockets transport, subprotocol, mgmt
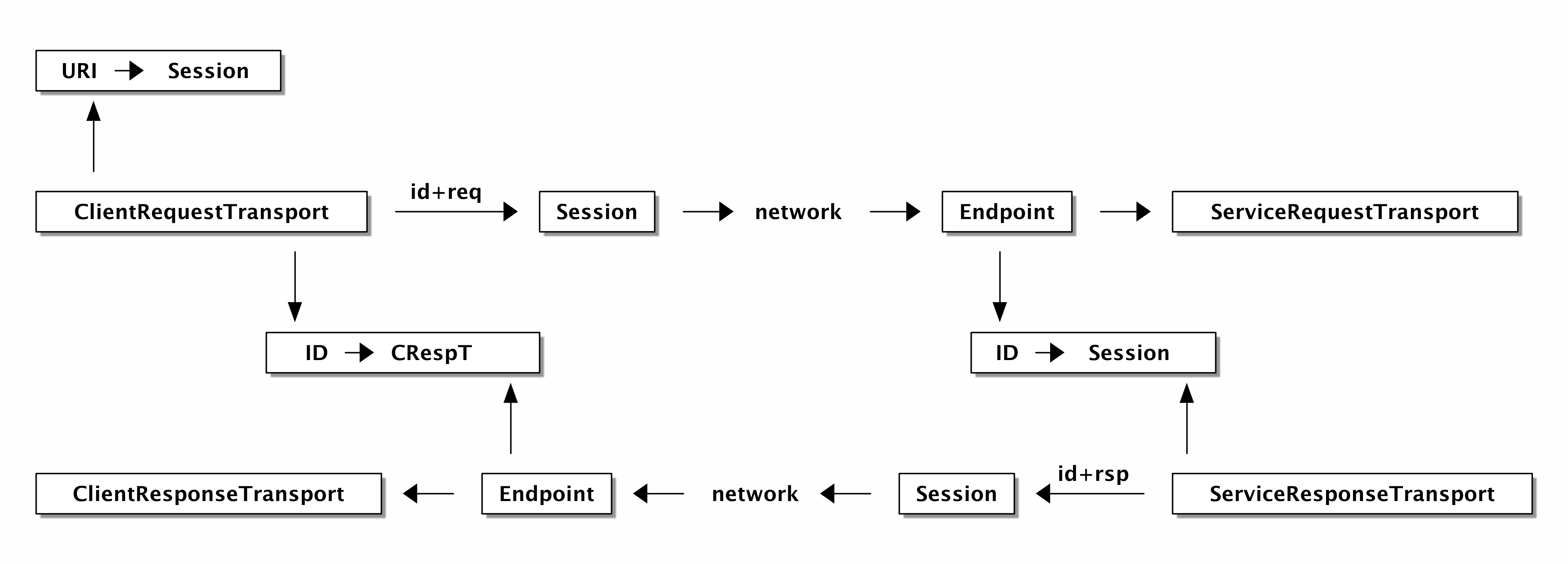
WebSockets code
demo
Using JSR-356 : JavaAPI for WebSocket
MS-SWSB : SOAP Over WebSocket Protocol Binding Specification
<wsdl:definitions ...> ...
<wsdl:binding name="MyBinding" type="MyPortType"> ...
<soap12:binding
transport="http://schemas.microsoft.com/soap/websocket"/>
<wsdl:operation name="MyOp"> ... </wsdl:operation>
</wsdl:binding>
<wsdl:service name="MyService">
<wsdl:port name="MyPort" binding="MyBinding">
<soap12:address location=" ws://myHost/myService/" />
</wsdl:port>
</wsdl:service>
</wsdl:definitions>
MS-SWSB : SOAP/WebSocket protocol example
GET http://myHost/myService HTTP/1.1
Connection: Upgrade,Keep-Alive
Upgrade: websocket
Sec-WebSocket-Key: ROOw9dYOJkStW2nx5r1k9w==
Sec-WebSocket-Version: 13
Sec-WebSocket-Protocol: soap
soap-content-type: application/soap+msbinsession1
microsoft-binary-transfer-mode: Buffered
Accept-Encoding: gzip, deflate
HTTP/1.1 101 Switching Protocols
Upgrade: websocket
Connection: Upgrade
Sec-WebSocket-Accept: s3pPLMBiTxaQ9kYGzzhZRbK+xOo=
Sec-WebSocket-Protocol: soap
InfiniBand transport
why InfiniBand
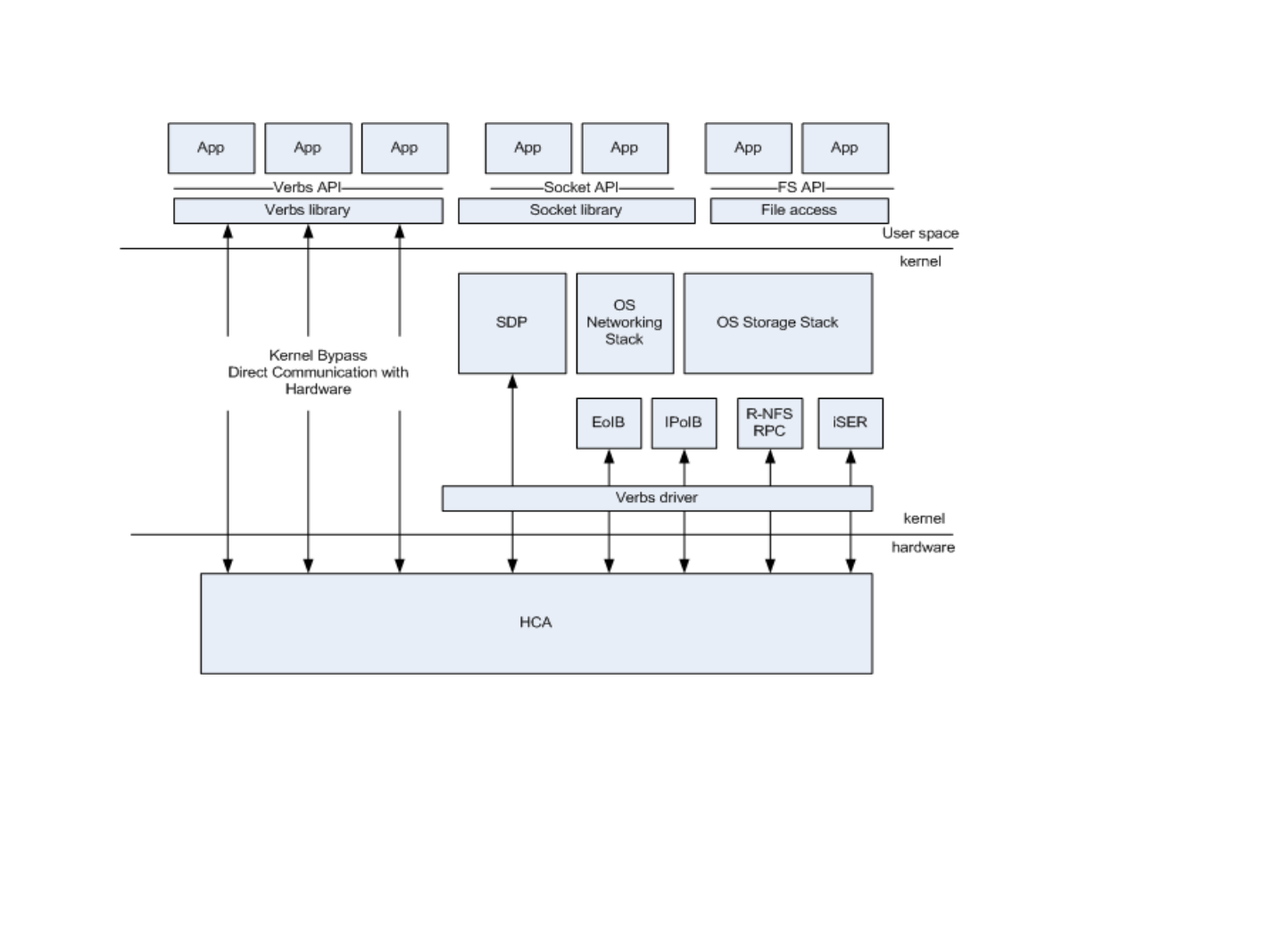
InfiniBand communications model
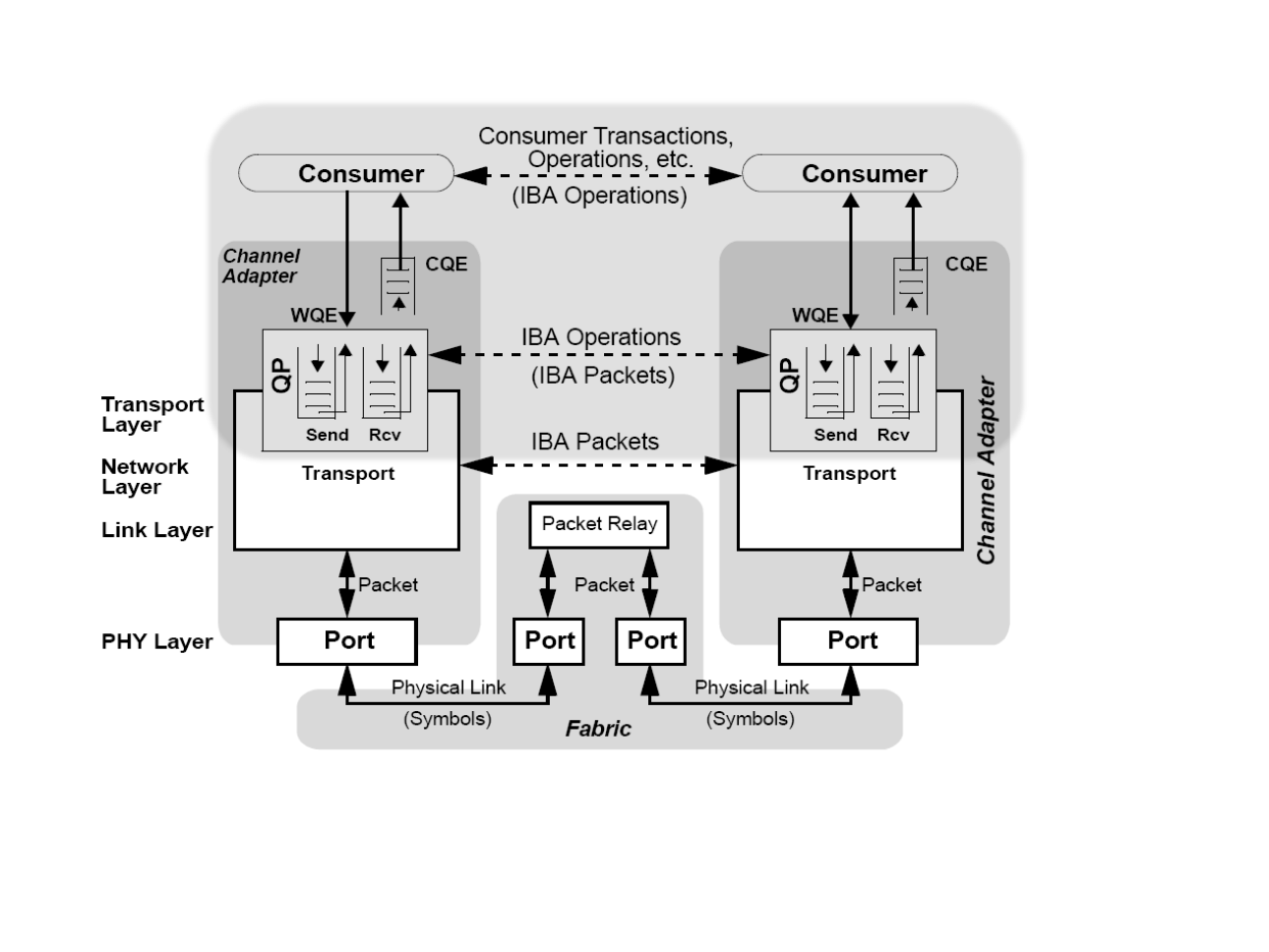
InfiniBand queue-pair model
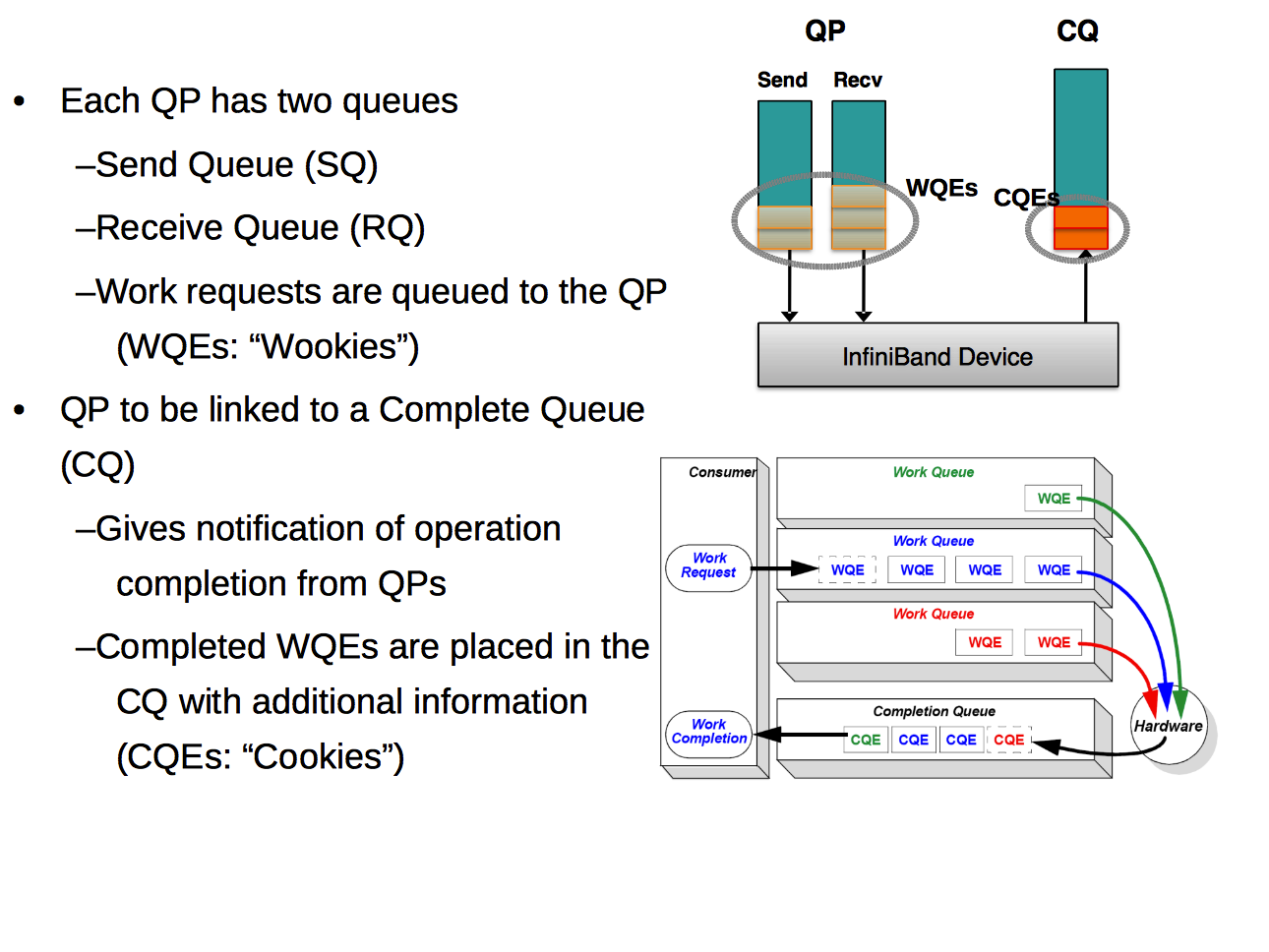
InfiniBand usage
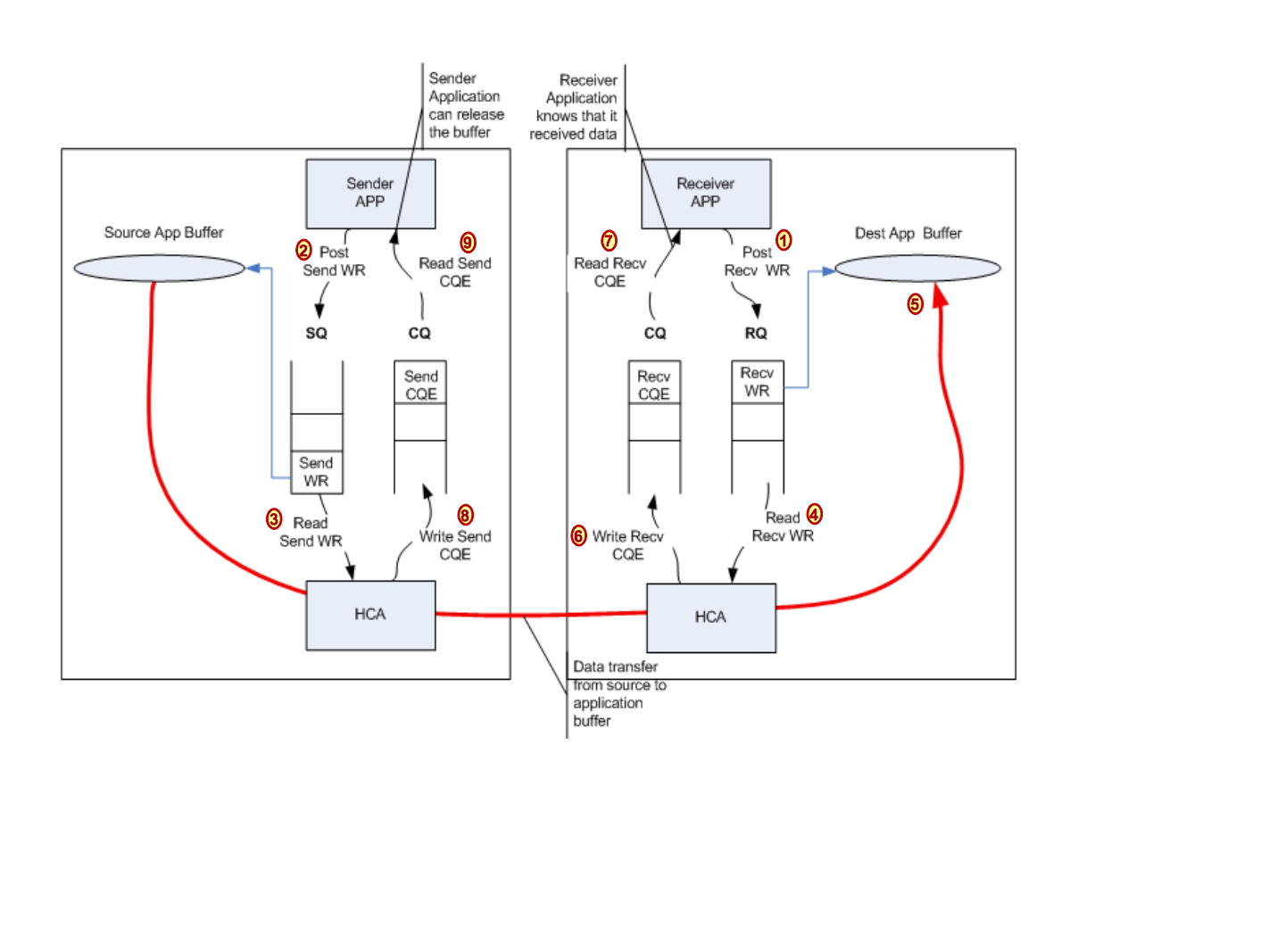
InfiniBand code
demo
Using proprietary JavaAPIs on top of proprietary C APIs.
InfiniBand credits
InfiniBand slides taken from
Vadim Makhervaks (Oracle)
http://www.ics.uci.edu/~ccgrid11/files/ccgrid11-ib-hse_last.pdf
DISI major interfaces
DISI client factories
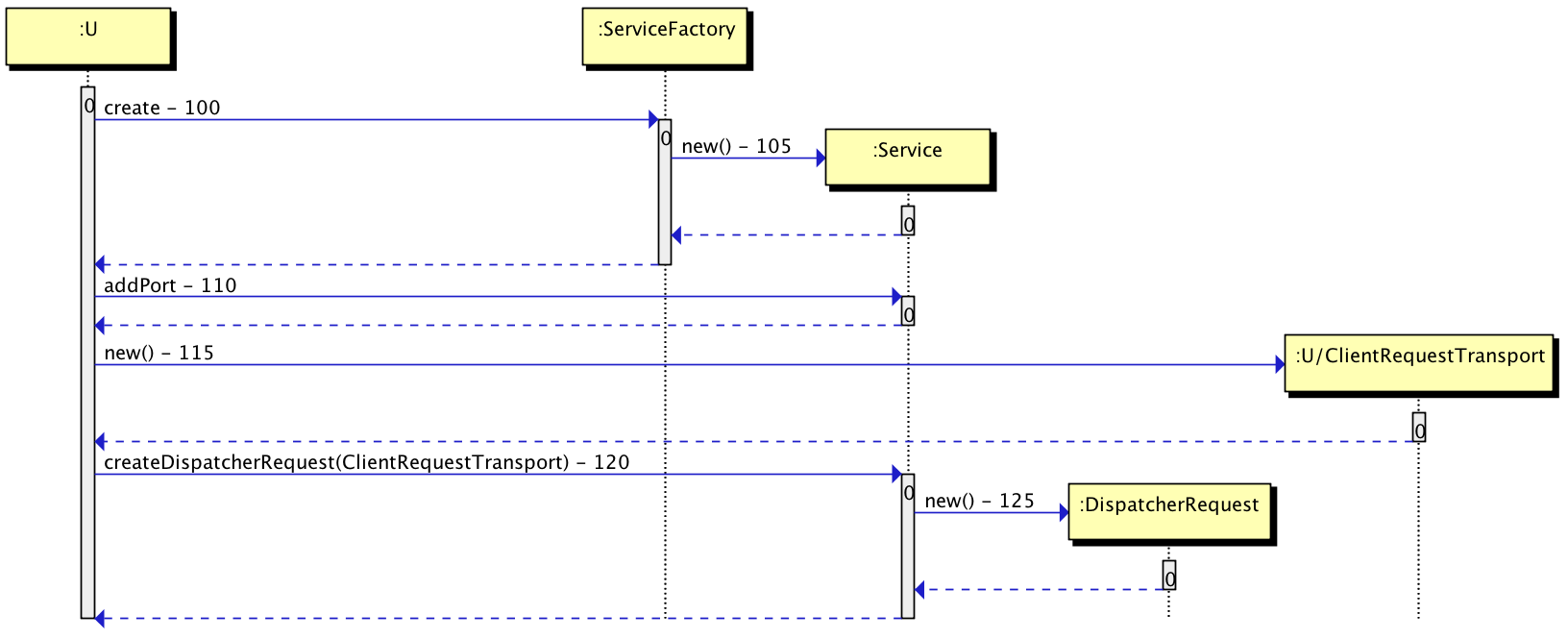
DISI service factories
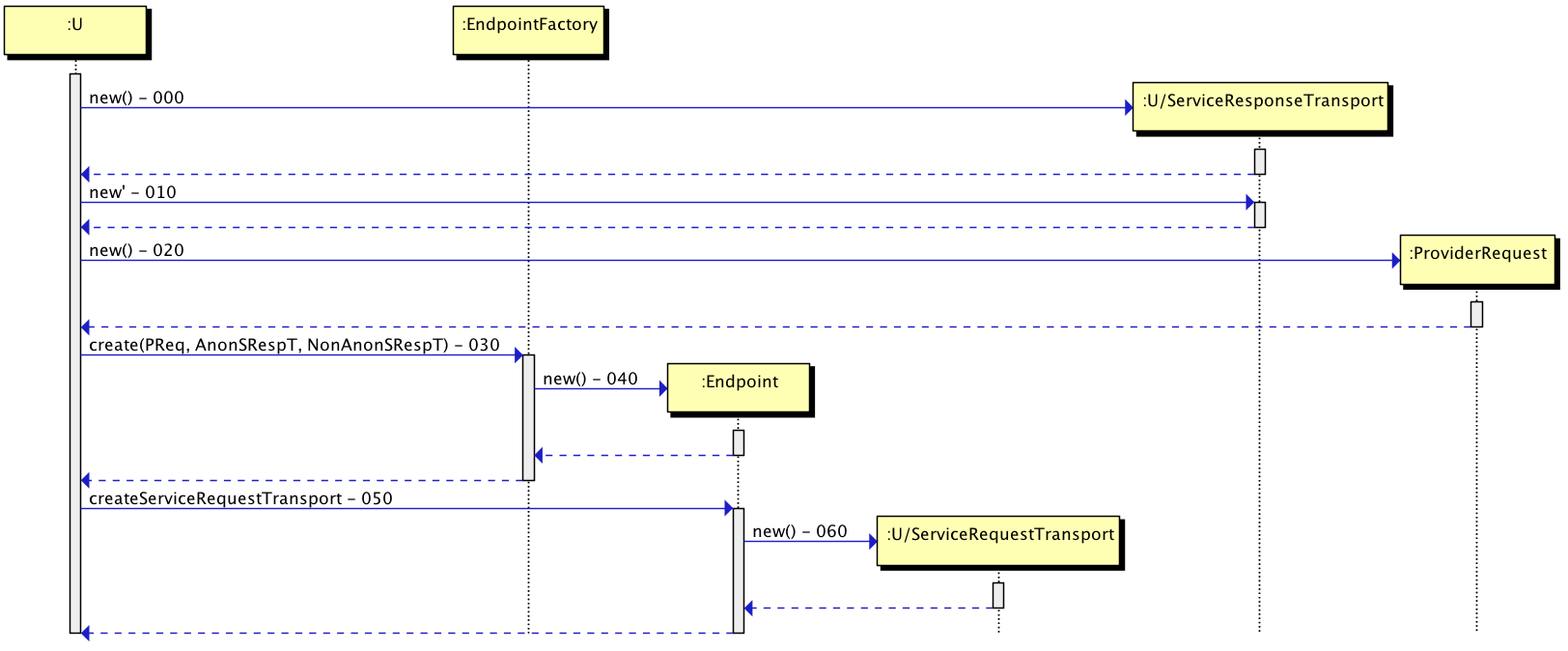
DISI client request (+ MessageContext factory)
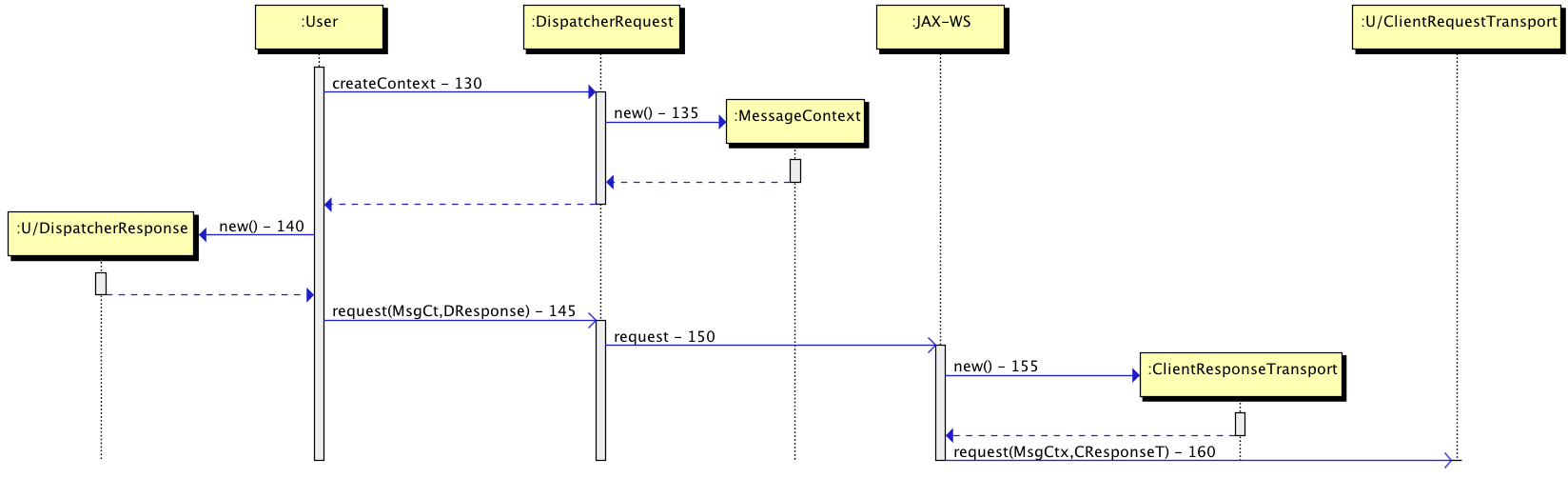
DISI service request (+ MessageContext factory)
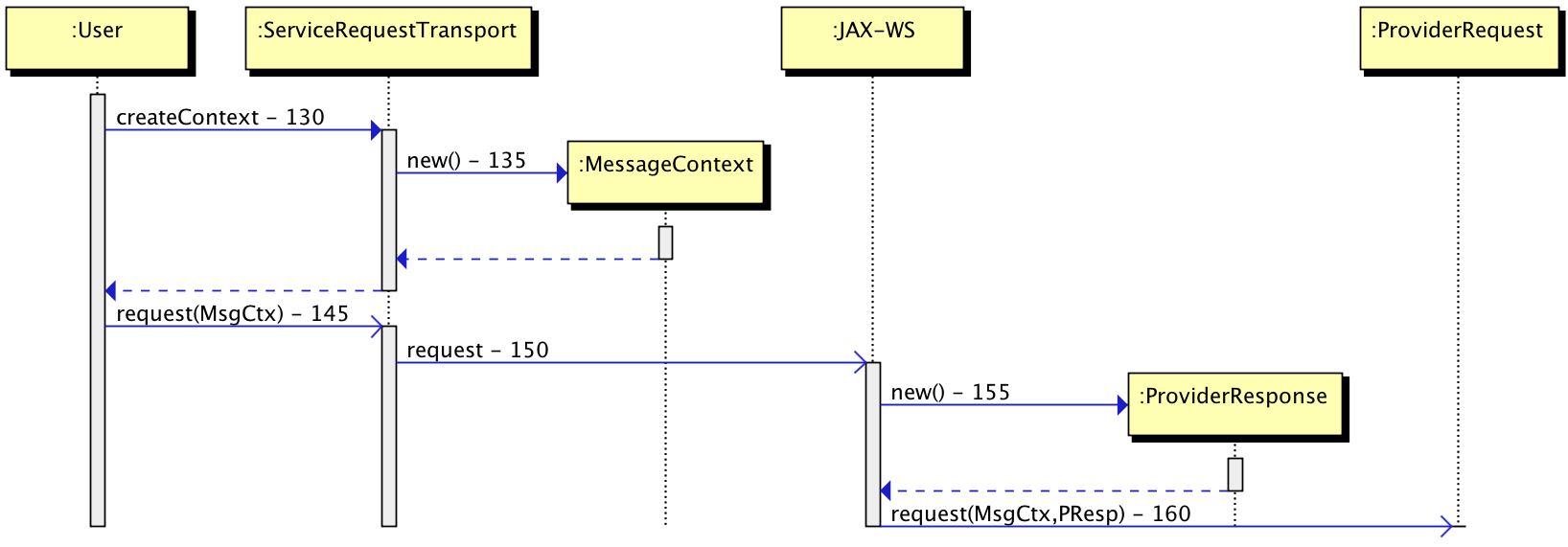
DISI client response (+ MessageContext factory)
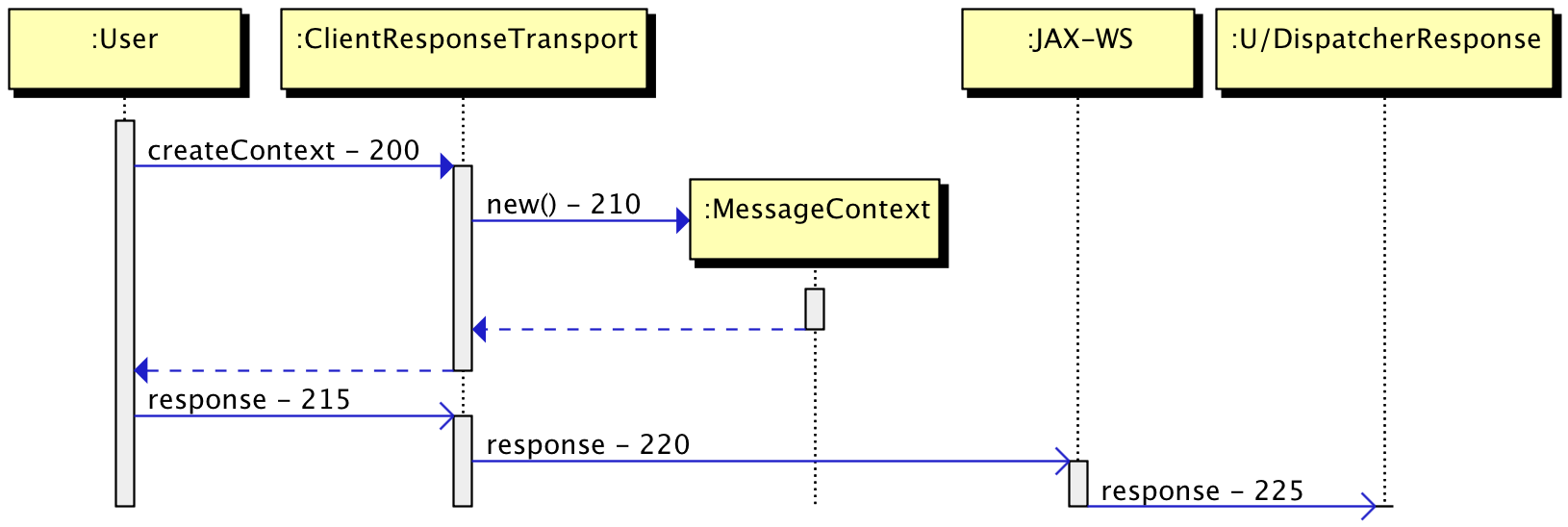
DISI service response (+ MessageContext factory)
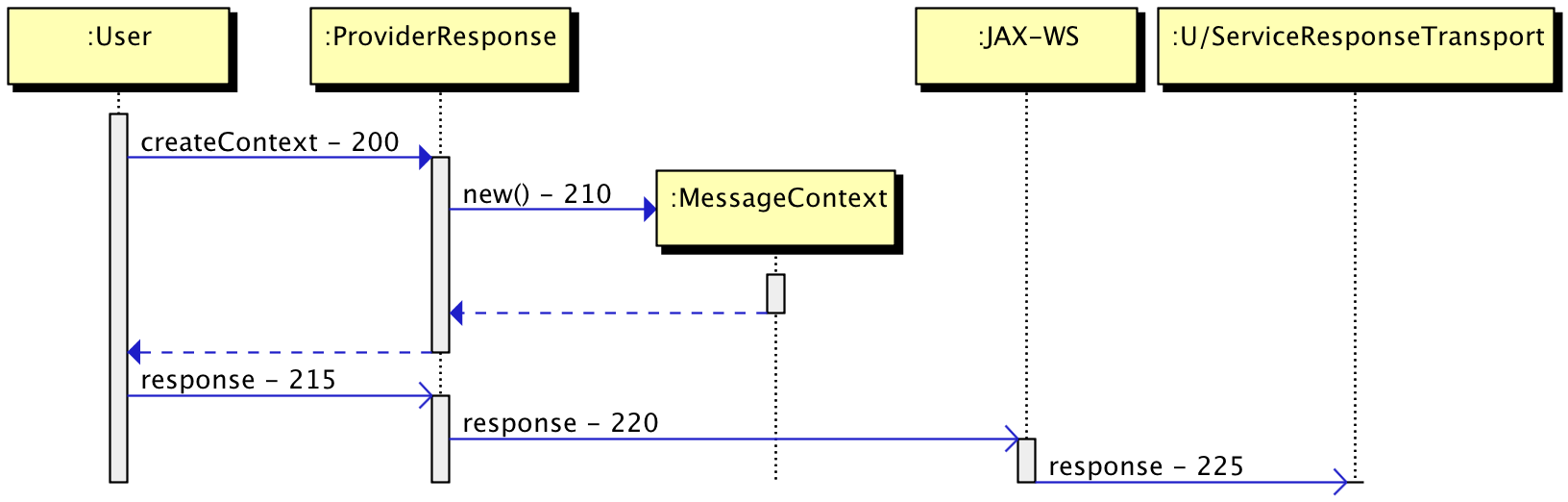
DISI MessageContext
/ Factory
public interface MessageContextFactory {
MessageContext createContext();
MessageContext createContext(SOAPMessage m);
MessageContext createContext(Source m);
MessageContext createContext(Source m,O EnvelopeStyle.Style envelopeStyle);
MessageContext createContext(InputStream in,
String contentType) throws IOException;
}
public interface MessageContext {
SOAPMessage getAsSOAPMessage() throws SOAPException;
SOAPMessage getAsSource();
ContentType writeTo(OutputStream out) throws IOException;
ContentType getContentType();
Object get(Object k);
void put(Object k, Object v);
}
summary
summary
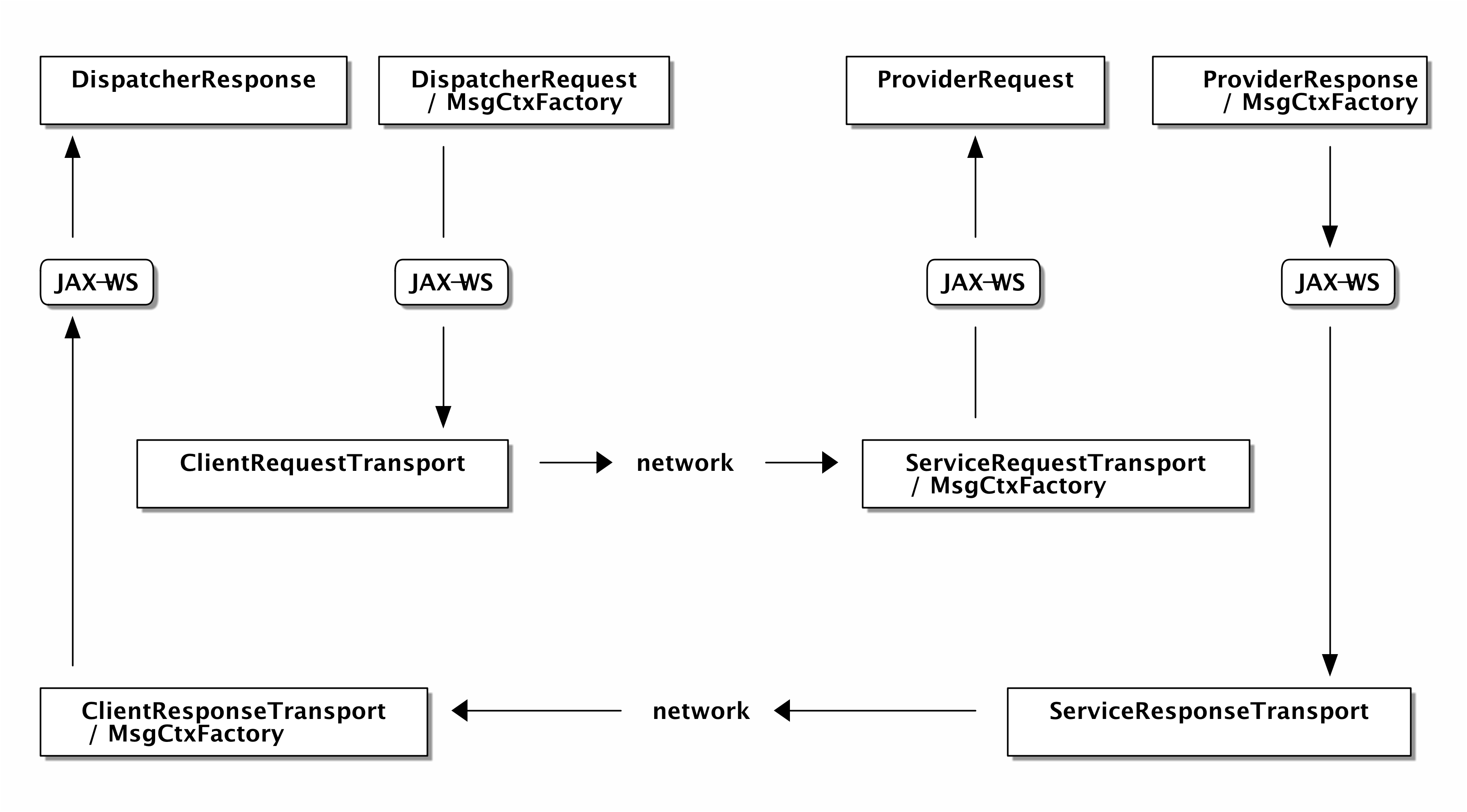
table of contents (non-functional)
- 1. me, disclaimer, copyright
- 2. intro
- 3. programming with existing JAX-WS
- 4. Dynamic Invocation and Service Interfaces (DISI)
- 5. WebSockets transport
- 6. InfiniBand transport
-
7. DISI major interfaces
- 7.1. DISI client factories
- 7.2. DISI service factories
- 7.3. DISI client request (+ MessageContext factory)
- 7.4. DISI service request (+ MessageContext factory)
- 7.5. DISI client response (+ MessageContext factory)
- 7.6. DISI service response (+ MessageContext factory)
-
7.7. DISI
MessageContext
/Factory
- 8. summary